Websocket
Websocket是Html5的一種網路協定,用於前端瀏覽器(Client)與後端(Server)的溝通,協定只需要連線一次,除非有一方斷開連接,否則就會一直存在,不需重複請求,常用實作於聊天室、訊息推播、共同編輯上。
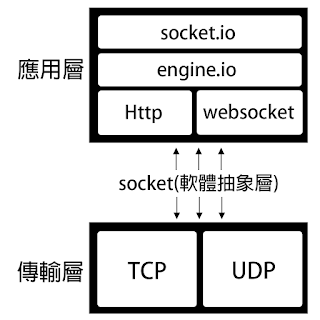
Socket.IO 一套 JavaScript 函式庫工具包
當時一些瀏覽器還不支持websocket協議的時候。socket.io會改選擇使用傳統的long polling方式運作。如果支援的話則會用websocket的協議。
安裝 socket IO 和 socket IO Client版
socket.io:node.js http server 使用
socket.io-client: client 瀏覽器端 使用
安裝指令:
npm i -D socket.io socket.io-client
使用方法
Server 端 |
建立連線/事件傳送方向 |
Client 端 |
io.on(‘connection’, (socket) => {…}) |
建立連線 |
socket = io(“socket ip:port”) |
io.emit(“要對 所有 Client 廣播的事件名稱”, data) |
———> |
socket.on(“來自client 的事件名稱”, callback) |
socket.emit(“要對 當前連線 的 Client 發送的事件名稱”, data) |
———> |
socket.on(“來自client 的事件名稱”, callback) |
socket.on(“來自client 的事件名稱”, callback) |
<——— |
socket.emit(“要對 server 發送的事件名稱”,data) |
Cheatsheet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| socket.emit('message', "this is a test");
socket.broadcast.emit('message', "this is a test");
socket.broadcast.to('game').emit('message', 'nice game');
socket.to('game').emit('message', 'enjoy the game');
socket.broadcast.to(socketID).emit('message', 'for your eyes only');
io.emit('message', "this is a test");
io.in('game').emit('message', 'cool game');
io.of('myNamespace').emit('message', 'gg');
socket.emit();
socket.broadcast.emit();
socket.on();
io.sockets.socket();
io.sockets.emit();
io.sockets.on() ;
|
Server端
如何建立連接
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import express from 'express'; import { createServer } from 'node:http'; import { fileURLToPath } from 'node:url'; import { dirname, join } from 'node:path'; import { Server } from 'socket.io';
const app = express(); const server = createServer(app); const io = new Server(server);
const __dirname = dirname(fileURLToPath(import.meta.url));
app.get('/', (req, res) => { res.sendFile(join(__dirname, 'index.html')); });
io.on('connection', (socket) => { console.log('a user connected'); });
server.listen(3000, () => { console.log('server running at http://localhost:3000'); });
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| io.on("connection", (socket) => { socket.emit('userID', socket.id);
socket.on("join", ({ userName, roomName }) => { const userData = userService.userDataInfoHandler( socket.id, userName, roomName ); userService.addUser(userData); socket.join(userData.roomName);
socket.broadcast.to(userData.roomName).emit("join", `${userData.userName} joined ${userData.roomName}`); });
socket.on("chat", (msg) => { const time = moment.utc(); const userData = userService.getUser(socket.id); if(userData){ io.to(userData.roomName).emit("chat", {userData, msg, time}); } });
socket.on("disconnect", () => { const userData = userService.getUser(socket.id); if (userData?.userName) { socket.broadcast.to(userData.roomName).emit("leave", `${userData.userName} left ${userData.roomName}`); } userService.removeUser(socket.id); }); });
|
Client端
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| import { io } from "socket.io-client";
const clientIo = io();
clientIo.emit("join", { userName, roomName });
clientIo.on("join", (msg: string) => { roomMsgHandler(msg); });
submitBtn.addEventListener("click", () => { const textValue = textInput.value; clientIo.emit("chat", textValue); });
clientIo.on("chat", (data) => { msgHandler(data); });
clientIo.on("leave", (msg: string) => { roomMsgHandler(msg); });
clientIo.on("userID", (id) => { userID = id; });
|
參考資料